Beberapa Tip untuk Para Pengguna JQuery Tingkat Lanjut
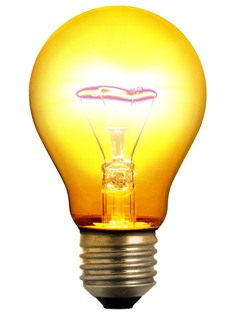
Berikut ini adalah beberapa tip penggunaan jQuery tingkat lanjut untuk Anda yang menginginkan performa web yang lebih baik, entah itu dari segi lama/cepatnya waktu eksekusi skrip ataupun dari segi panjang/pendeknya penulisan kode. Semoga bermanfaat!
Kode Pintasan untuk ‘DOM Ready’
// Sebelum
$(document).ready(function() {
…
});
// Sesudah
$(function() {
…
});
Metode Berantai
Jika memang masih bisa dikaitkan, maka Anda sebenarnya tidak perlu menuliskan selektor jQuery berulang-ulang dan kemudian menerapkan metode pada selektor tersebut:
// Sebelum
$('button').click(function() {
$(this).toggleClass('active');
$(this).html('Clicked!');
$(this).css('opacity', .5);
});
$('button').css('color', 'red');
// Sesudah
$('button').click(function() {
$(this).toggleClass('active').html('Clicked!').css('opacity', .5);
}).css('color', 'red');
Menyeleksi Elemen <body>
dan <html>
Selektor ini akan memberikan performa yang lebih baik:
// Sebelum
var $root = $('html'),
$base = $('body');
// Sesudah
var $root = $(document.documentElement),
$base = $(document.body);
Mengambil/Mengeset Atribut Elemen dengan JavaScript Mentah
Dalam kondisi tertentu, metode ini terbukti jauh lebih efisien jika dibandingkan dengan metode .attr()
:
// Sebelum
$('div').each(function(i) {
$(this).attr({
'class': 'foo',
'id': 'bar-' + i
});
});
// Sesudah
$('div').each(function(i) {
this.className = 'foo';
this.id = 'bar-' + i;
});
// Sebelum
$('a').click(function() {
alert($(this).attr('href'));
});
// Sesudah
$('a').click(function() {
alert(this.href);
});
Menerapkan Metode JavaScript Mentah kepada Selektor jQuery
Anda bisa menggunakan jQuery .get()
untuk mengembalikan status selektor jQuery menjadi selektor JavaScript, atau gunakan indeks array yang ada untuk menyeleksi elemen pada urutan tertentu:
// Tidak valid
$('div').innerHTML = 'test';
// Valid
$('div').get(0).innerHTML = 'test';
$('div')[0].innerHTML = 'test';
// Tidak valid
$('#my-button').onclick = function() {
alert('test!');
};
// Valid
$('#my-button').get(0).onclick = function() {
alert('test!');
};
$('#my-button')[0].onclick = function() {
alert('test!');
};
Fungsi Anonim untuk Menggantikan .each()
// Sebelum
$('div').each(function(i) {
$(this).html('Element: ' + i);
});
// Sesudah
$('div').html(function(i) {
return 'Element: ' + i;
});
Mengeset Event dan Mengeksekusinya Secara Bersamaan
// Sebelum
function windowSizeCheck() {
$('#result').html('Width: ' + $(window).width() + '<br>Height: ' + $(window).height());
} windowSizeCheck();
$(window).resize(windowSizeCheck);
// Sesudah
$(window).resize(function() {
$('#result').html('Width: ' + $(this).width() + '<br>Height: ' + $(this).height());
}).trigger("resize");
Jalan Pintas untuk Efek Toggle
Setiap metode dalam jQuery pada umumnya adalah berupa objek, sehingga Anda bisa menganggap $(document).ready()
dan $(document)['ready']()
sebagai dua hal yang sama:
// Tidak efisien
$('a').mouseenter(function() {
$(this).addClass('hover');
});
$('a').mouseleave(function() {
$(this).removeClass('hover');
});
// Lebih efisien
$('a').mouseenter(function() {
$(this).addClass('hover');
}).mouseleave(function() {
$(this).removeClass('hover');
});
// Lebih efisien lagi
$('a').hover(function() {
$(this).addClass('hover');
}, function() {
$(this).removeClass('hover');
});
// Paling efisien
$('a').on("mouseenter mouseleave", function(e) {
$(this)[e.type == "mouseenter" ? 'addClass' : 'removeClass']('hover');
});
Efek Tabulasi
Gunakan .siblings()
untuk menyeleksi semua elemen yang sejenis dengan X di dalam induk yang sama tanpa menyeleksi elemen X itu sendiri:
/**
* <div class="my-tab-buttons">
* <a href="#tab-1">Tab 1</a>
* <a href="#tab-2">Tab 2</a>
* <a href="#tab-3">Tab 3</a>
* <a href="#tab-4">Tab 4</a>
* </div>
*/
// Sebelum
$('.my-tab-buttons a').click(function() {
$(this).parent().find('a').removeClass('active');
$(this).addClass('active');
return false;
});
$('.my-tab-buttons a:first').addClass('active');
// Sesudah
$('.my-tab-buttons a').click(function() {
$(this).addClass('active').siblings().removeClass('active');
return false;
}).first().trigger("click");
Efek Animasi Beruntun
Sebelumnya Saya sudah pernah menjelaskan tentang ini…
// Sebelum
$('div')
.animate({'font-size':10}, 400)
.animate({'font-size':50}, 400)
.animate({'font-size':100}, 400)
.animate({'margin-top':200}, 400);
// Sesudah
var anim = [
{'font-size':10},
{'font-size':50},
{'font-size':100},
{'margin-top':200}
];
$.each(anim, function(i) {
$('div').animate(anim[i], 400);
});
// Sebelum
$('div')
.animate({'font-size':10}, 400)
.animate({'font-size':50}, 1000)
.animate({'font-size':100}, 200)
.animate({'margin-top':200}, 300);
// Sesudah
var anim = [
[{'font-size':10}, 400],
[{'font-size':50}, 1000],
[{'font-size':100}, 200],
[{'margin-top':200}, 300]
];
$.each(anim, function(i) {
$('div').animate(anim[i][0], anim[i][1]);
});
Efek Berdenyut
function pulsate() {
var $div = $('div');
$div.animate({
fontSize: $div.css('font-size') == '50px' ? '100px' : '50px'
}, 800, pulsate);
} pulsate();
Labels: JavaScript, jQuery, Lanjutan